Prerequisites
- Products: Liquid UI WS, Liquid UI Server or Local DLL, Client Software
- Commands: del(), load(), pushbutton(), message()
- File: wsoffice.dll
Purpose
Learn how to open the desired excel file with a pushbutton created on an SAP screen. To demonstrate this, we'll guide you through the following steps.
- Delete unnecessary elements on the SAP using the del command
- Add a pushbutton to open the pop-up window on click
- Create a function to select the excel file
- Create a function to open the desired excel file
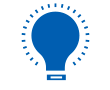
Note: You need to place the wsoffice.dll file in your scripts folder and it must be loaded in the ESESSION.SJS file.
User Interface
// Create this file inside your script folder for customizing the SAP Easy Access screen SAPLSMTR_NAVIGATION.E0100.sjs
//Now, let's start adding the Liquid UI script to the above file and save it.
Customization
- Logon to SAP and delete the image container on the SAP Easy Access screen, as shown in the image below:
// Deletes an image container on the SAP Easy Access screen del("X[IMAGE_CONTAINER]");
Screen Script
- Add a toolbar pushbutton with the label Open Excel File to execute the process called SelectExcelfile and open a pop-up window on click.
// Creates a pushbutton with the label Open Excel File to open a pop-up when clicked. pushbutton([TOOLBAR],"@48@Open Excel File", "?", {"process":SelectExcelfile});
- Use the load command to add the wsoffice to the SAPLSMTR_NAVIGATION.E0100.sjs file; this allows you to access the functions included in it.
// Need to load this file to display the File Selection pop-up load('wsoffice');
- Now, add the following Liquid UI script to the file and save it.
//function SelectExcelfile(param){
if(szPrompt==void 0) szPrompt = 'Select the Excel File';
var dialog = new ActiveXObject('MsComDlg.CommonDialog');
dialog.Filter='Excel Files(*.xlsx*)|*.xlsx*';
dialog.MaxFileSize=32767;
dialog.DialogTitle=szPrompt;
dialog.Flags=0x200|0x80000|0x800|0x4|0x200000
dialog.ShowOpen();
var ret = dialog.FileName;
dialog = void 0;
if(file!=""){
ifopenExcelfile(file)
}
else{
message("File Not Selected!",{"StatusLine":true,"title":"Information","type":"W"});
}
}
// Function to Open the Excel File
function openExcelfile(filename){
g_ExcelApp = new ActiveXObject('Excel.Application');
g_ExcelBook=g_ExcelApp.Workbooks.Open(filename);
g_ExcelApp.Visible=true;
g_ExcelApp.ScreenUpdating=true;
message("S:" +filename+" Excel File is Opened Successfully");
}
SAP Process
- Now, refresh the SAP screen, and click on the Open Excel File toolbar pushbutton. Then appears a pop-up window, as shown below.
- Select the excel file that you need to open and click on Open, as shown in the following image.
- Then, the selected excel file will be opened with a success message "Excel File is Opened Successfully," along with the file path, as shown in the image below.
- If no file is selected, an error message is displayed "File Not Selected," as shown in the following image.