Prerequisites
- Products: Liquid UI WS, Liquid UI Server or Local DLL, Client Software
- Commands: inputfield(), clearscreen(), pushbutton(), return()
Purpose
In this article, we will demonstrate the process of making a remote function call to display a message for transactions that require immediate acknowledgment of completion, indicating the success or failure of the RFC call. Here we are considering the SAP Easy Access screen as an example and we’ll guide you through the following steps.
- Delete the unnecessary elements on the SAP Easy Access screen
- Add an input field to display the message
- Add a push button to get the message
- Add functionality to remove blank spaces, return trimmed strings, check for blank strings, and display messages in the input field.
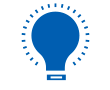
Note: Ensure to provide the RFC parameters in the guixt.sjs file.
User Interface
//Create the SAPLSMTR_NAVIGATION.E0100.sjs file inside your scripts folder for customizing the SAP Easy Access screen
//Now, let's add the Liquid UI script to the above file and save it
Customization
- Delete the unnecessary elements on the SAP Easy Access screen using the clearscreen command.
//Deletes the unnecessary elements on the SAP screen clearscreen();
- Add a read-only input field labeled Message to display the message upon a function call.
//Creates a read-only input field inputfield([1,0],"Message",[1,10],{"name":"z_message","size":70,"readonly":true});
- Add a push button labeled Get Message to display the message in the input field upon clicking.
//Creates a push button to execute getMessage process on click pushbutton([3,1],"Get Messsage","?",{"size":[2,20],"process":getMessage});
- Add a function to remove the blank spaces.
//Function to remove blank spaces from variable values String.prototype.trim = function() { return this.replace(/^\s+|\s+$/g,""); }
- Add a function getString to return the trimmed string.
//Function to return trimmed string function getString(strInput) { return (typeof(strInput) == 'undefined' || strInput == 'undefined') ? "" : strInput.toString().trim(); }
- Add the isBlank function to check for a blank string.
//Function to check for a blank string function isBlank(strInput) { var strVal = getString(strInput); var blank = strVal == ""; return blank; }
- Add a function to display the result in the input field after the RFC function execution.
//Function to call BAPI and display results getMessage() { onscreen 'SAPLSMTR_NAVIGATION.0100' rfcresult = call('BAPI_USER_CHANGE',{'in.USERNAME':'&V[_user]','out.GENERATED_PASSWORD':'z_gen_pswd','table.RETURN':'z_return'}); if(rfcresult.rfc_rc != 0) { // Cannot Call RFC for any reason // RFC call was *NOT* successful. message('E: Error! RFC Call Failed to Return Data'); enter("?"); goto SCRIPT_END; } else { if(!isBlank(rfcresult.exception)) { // RFC Call succeeded, but the ABAP code in the function module generated an exception // Display message to the user, that rfc exception occurred (you can use rfcresult.exception) message('E: Error! '+rfcresult.exception); enter("?"); goto SCRIPT_END; } } // RFC call was successful if(!isBlank(z_return)){ set('V[z_message]', z_return.toString().substring(24,244)); } message('S: RFC call was successful'); enter('?'); SCRIPT_END:; }
SAP Process
- Refresh the SAP screen, and click the Get Message button. Upon successful RFC execution, you'll see an RFC message in the Message field and a success message at the bottom.
- In the absence of an RFC invocation, an error message is displayed, as shown below.