Prerequisites
- Products: Liquid UI WS, Liquid UI Server or Local DLL, Client Software
- Commands: inputfield(), pushbutton(), set()
Purpose
You will learn how to remove the padded values from a string value entered in an input field. It is used to remove characters from the beginning or end of the string. To demonstrate this process, we are considering the SAP Easy Access screen as an example, and guide you through the following steps:
- Delete the image container on the SAP Easy Access screen.
- Add an input field where the user can enter the value and a non-editable input field to display the value with padded zeroes removed from either the left or right.
- Add two push buttons that execute the corresponding function and remove zeroes from the user-entered value.
- Add a function to remove the padded values from a source.
- Add a function to remove the padded values from the left.
- Add a function to remove the padded values from the right.
User Interface
//Create the file SAPLSMTR_NAVIGATION.E0100.sjs inside your scripts folder for customizing the SAP Easy Access screen
//Now, let's add the Liquid UI script to the above file and save it
Customization
- Delete the image container on the SAP Easy Access screen using the del command.
//Deletes the image container on the SAP screen del("X[IMAGE_CONTAINER]");
- Add an input field labeled Enter Value for users to enter a value, and a non-editable field labeled New Value to display the value with padded zeroes removed from either the left or right side.
//Creates an input field where the user can enter the values inputfield([1,0], "Enter Value", [1,16], {"size":10, "name":"z_value"}); //Creates an non-editable input field to display the value inputfield([2,0], "New Value", [2,16], {"size":10, "name":"z_unpadded_value", "readonly":true});
- Add two push buttons, labeled Remove Zeroes Left and Remove Zeroes Right, which execute the corresponding function upon click to remove zeroes from the user-entered value.
//Creates a push button with a label as Remove Zeroes Left and executes the removeZeroesLeft function on click pushbutton([4,0], "Remove Zeroes Left", {"process":removeZeroesLeft, "size":[1,18]}); //Creates a push button with a label as Remove Zeroes Right and executes the removeZeroesRight function on click pushbutton([4,20], "Remove Zeroes Right", {"process":removeZeroesRight, "size":[1,18]});
- Add the unPadZeroes function to remove zeroes padded to the source based on the direction specified.
//Function to remove zeroes padded to the source based on the direction specified function unPadZeroes(source,direction) { var output = ""; var sourceLength = 0; var sourceOriginalLength = 0; source = source.toString(); if(source) { sourceOriginalLength = source.length; sourceLength = source.length; } switch (direction){ case "LEFT": for (var loop = 0; loop < sourceOriginalLength; loop++) { if(source[0]=='0'){ source = source.substring(1,sourceLength); sourceLength = source.length; } else { break; } } break; case "RIGHT": for (var loop = 0; loop < sourceOriginalLength; loop++) { if(source[sourceLength-1]=='0'){ source = source.substring(0,sourceLength-1); sourceLength = source.length; } else { break; } } break; } return source; }
- Add the removeZeroesLeft function to remove zeroes padded on the left of the field value.
//Function to remove zeroes to the left of the source function removeZeroesLeft(){ z_temp = unPadZeroes(z_value,"LEFT"); set('V[z_unpadded_value]','&V[z_temp]'); return; }
- Add the removeZeroesRight function to remove zeroes padded on the right of the field value.
//Function to remove zeroes to the right of the source function removeZeroesRight(){ z_temp = unPadZeroes(z_value,"RIGHT"); set('V[z_unpadded_value]','&V[z_temp]'); return; }
SAP Process
- Refresh the SAP screen and enter the value into the Enter Value input field. Clicking on the Remove Zerores Left push button removes the zeroes padded to the value entered and displays the value in the New Value input field.
- Similarly, clicking on the Remove Zeroes Right push button removes zeroes padded to the right and displays the value in the New Value input field.
Next Steps
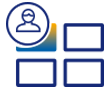
Return values from new session
Learn how to return values from one session to another session.
Learn how to return values from one session to another session.
10 min.
This article is part of the Invoking functions tutorial.